Hello everyone! So this article will be on how you can create your own Docker registry hub and push your own Docker images to it. So before we start, here are the prerequisites. They're obvious, but let's make sure.
How The Application works within this website
Posted on April 13, 2019461 views7 min read
Hey everyone! So I guess I'll further explain in details how strict my MVC application is. I am a pretty strict OOP user. I see MVC as 3 different entities. # Controller The Controller file(s) *should only* be dealing with what to do next based on the action. They should not be outputting to the screen. That is the task of the View. It makes no sense to be outputting in this case. The Controller file(s) also should not be hard coding or making any database calls. That is the task of the Model. If you want to make database calls, do that in the Model file(s) and return the results back to the Controller. # Model The Model file(s) *should only* be dealing with database calls. They *should not* be outputting to the screen. That is the task of the View. It makes no sense to be outputting in this case. # View The View file(s) *should only* be dealing with outputting to the screen. They *should not* be making any database calls. That is the task of the Model.
So each part has its own purpose. It makes no sense to swap the roles around because someone feels lazy. The View should not know that the Model exists and vice versa. The only entity that should know that both exists are the Controllers. The Controllers dictate what each page should have so it makes sense for the Controllers to have access to both Views and Models. Here is how I view MVC.
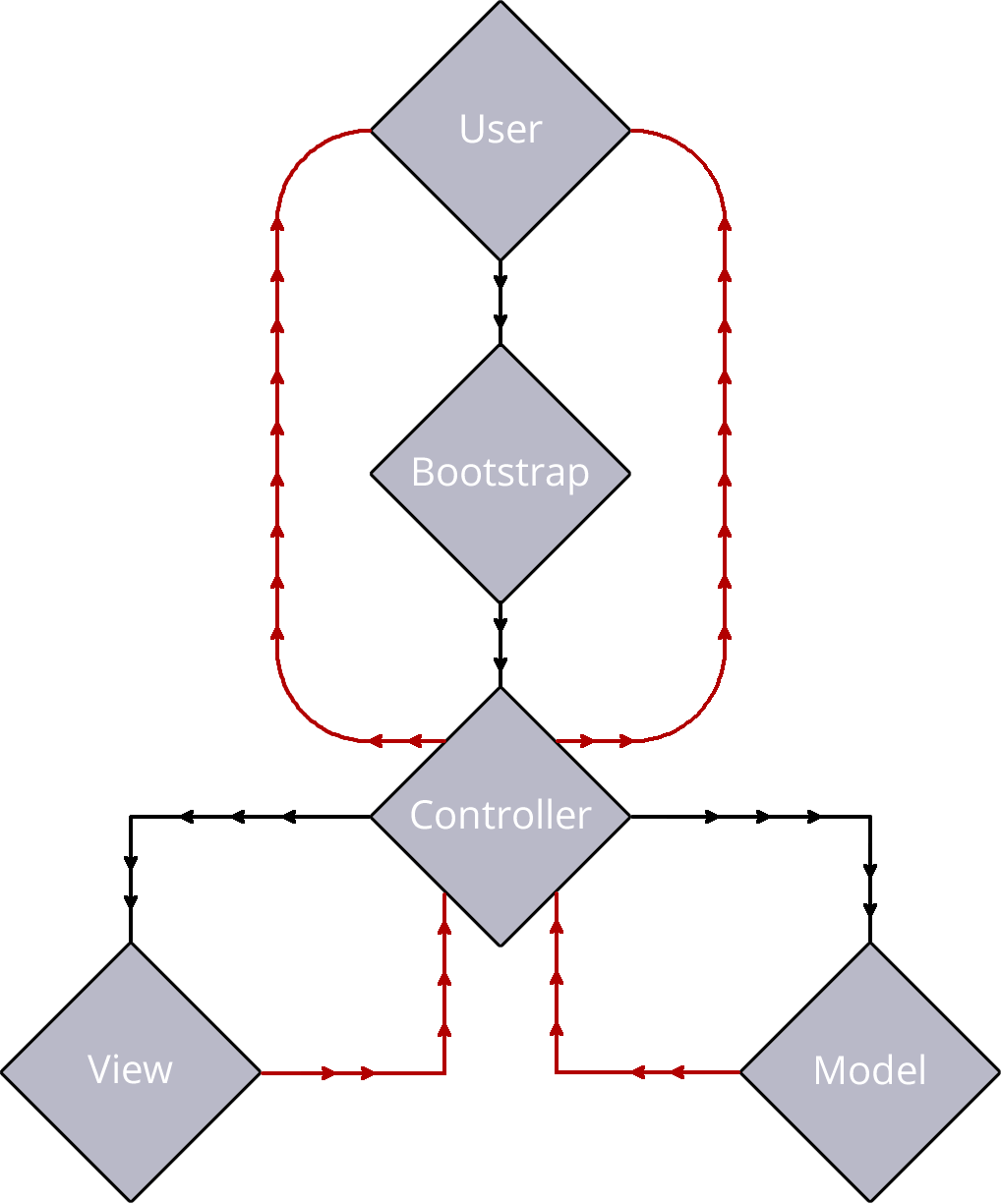
$this->call->controller('controller_name', 'methodName', ['optionalArgument' => $optionalArgument]);
There is only 3 arguments that you need to pass within the controller's call.
- The first argument is always the controller name. You don't need to use the full path.
- The second argument is always the method name. The default method will be always be index().
- The third argument is optional and you do not need to pass this argument unless you are passing around variables to other controllers. This argument is an array.
## Model
$this->model->modelClass->methodClass();
To load up your custom model, you will have to adhere to the naming conventions within this application. The filename has to end in .class.php as well as the class name has to be TestClass where Test is the model filename and Class is the .class part from the filename. Together it becomes TestClass. Another example would be ExampleClass where the filename is example.class.php.
To then call your custom model, you will have to call your class like so $this->model->testClass or $this->model->exampleClass. This will load up your custom model class. After doing so, you just need to call the method name. In the full example above, the method name is methodClass(). If the method name requires variables to be passed in the argument, it would look something like so.
$this->model->modelClass->methodClass($arg1, $arg2, $arg3);
Where $arg1, $arg2, and $arg3 are variables from your controller file.
## View
$passableVariable = 'Hello World!';
$this->render->view('view_file', [
'passableVariable' => $passableVariable
]);
To use the render class, you only need 2 arguments.
- The first argument is always the view file. Again, you do not need to use the full path.
- The second argument is an array and it's your passable variables.
It is simple to use MVC within this application. The final example use is below. # Controller
<?php
namespace The\Application;
if(!defined('CHECK')) {
require_once '../../core/rheta.php';
$rheta = new \The\Application\Rheta();
die;
}
use \The\Application\Controller;
class Test extends Controller {
public function __construct() {
parent::__construct();
}
public function index() {
$testMethod = $this->model->testClass->testMethod();
if($testMethod == false) {
$this->call->controller('404', 'index');
} else {
$this->render->view('test', [
'passableVariable' => $testMethod
]);
}
}
}
# Model
<?php
namespace The\Application\Model;
if(!defined('CHECK')) {
require_once '../core/rheta.php';
$rheta = new \The\Application\Rheta();
die;
}
use \PDO;
use \PDOException;
use \stdClass;
class TestClass {
public function __construct($db) {
try {
$this->db = $db;
} catch (PDOException $e) {
die('Database connection could not be established.');
}
}
public function testMethod() {
$sql = 'SELECT id, name FROM users';
$prepare = $this->db->prepare($sql);
$prepare->execute();
if($prepare->rowCount()) {
return $prepare->fetchAll();
} else {
return false;
}
}
}
# View
<?php
print_r($passableVariable);
It is that simple.
Another limitation that I have set is that you cannot use include() or require() if you want to include a file. You have to call it the correct way. If you want to include a controller, then use $this->call->controller();. If you want to include a view, then use $this->render->view();. If you want to include a model, then use $this->model->.... Running either include() or require() is incorrect because the 3 ways above should be sufficient enough.
Here is an 8 minute video demonstrating how strict my application is.
Other limitations include running the asterisk (\*) wild card in your model queries. You should already know which columns to pick. You shouldn't have the need to run the asterisk (\*) wild card. So you will receive and error as well if you use the asterisk (\*) wild card.